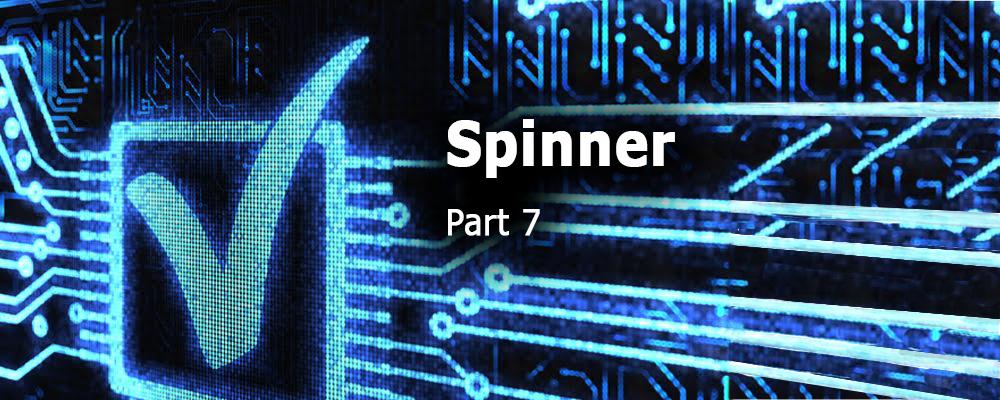
Creating QML Controls From Scratch: Spinner
Continuing our QML Controls from Scratch series, this time we will implement a Spinner, also known as a busy indicator. Spinner indicates the progress of a long-running operation when the progress percentage is unknown. (For known percentages, we'd use a ProgressBar.) Spinner uses a Timer to animate rotation. Normally, a designer would provide us with a .png asset to rotate an Image, but for this example we rotate an array of circles, implemented with Rectangle. Spinner has no public properties.
Spinner.qml
import QtQuick 2.0
Item {
id: root
// public
// private
width: 500; height: 100 // default size
Item { // square
id: square
anchors.centerIn: parent
property double minimum: Math.min(root.width, root.height)
width: minimum; height: minimum
Repeater {
id: repeater
model: 8
delegate: Rectangle{
color: 'black'
property double b: 0.1
property double a: 0.25
width: ((b - a) / repeater.count * index + a) * square.height; height: width
radius: 0.5 * height
x: 0.5 * square.width + 0.5 * (square.width - width ) * Math.cos(2 * Math.PI / repeater.count * index) - 0.5 * width
y: 0.5 * square.height - 0.5 * (square.height - height) * Math.sin(2 * Math.PI / repeater.count * index) - 0.5 * height
}
}
}
Timer {
interval: 10
running: true
repeat: true
onTriggered: square.rotation += 2 // degrees
}
}
Test.qml
import QtQuick 2.0
Spinner{}
Summary
In this post, we created a Spinner. Use it to show progress of a long-running operation in cases where you don't know the progress percentage. In the next blog, we'll create a Dialog. The source code can be downloaded here. Be sure to check out my webinar on-demand. I walk you through all 17 QML controls with code examples.