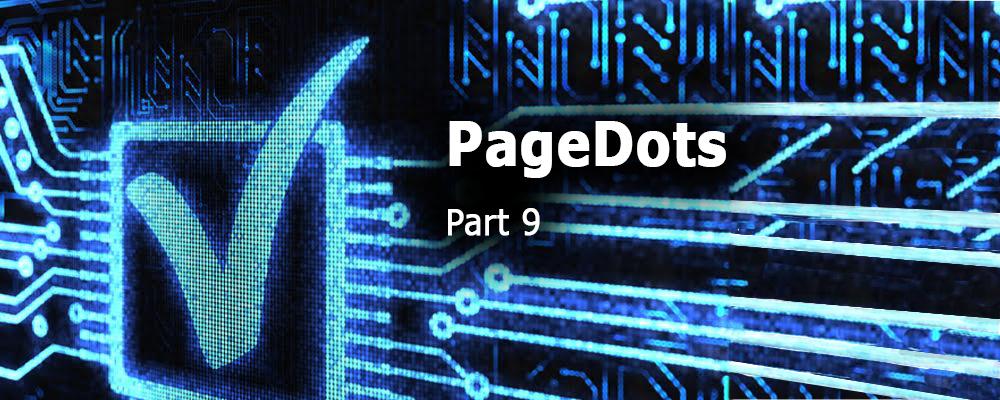
Creating QML Controls From Scratch: PageDots
Continuing our QML Controls from Scratch series, this time we implement PageDots. They are often used in conjunction with ListView.SnapOneItem to indicate what "page" is currently shown.
The public properties are page and pages to indicate the current page and total number of pages respectively. The dots are implemented with a Rectangle (configured as a circle) inside a Repeater.
PageDots.qml
import QtQuick 2.0
Item {
id: root
// public
property int page: 0 // current
property int pages: 3 // total
// private
width: 500; height: 100 // default size
Row {
spacing: (root.width - pages * root.height) / (pages - 1)
Repeater {
model: pages
delegate: Rectangle { // circle
width: root.height; height: width
radius: 0.5 * width
color: index == page? 'black': 'white'
border.width: 0.05 * root.height
}
}
}
}
Test.qml
import QtQuick 2.0
ListView { // PageDots
id: listView
width: 250; height: 100
snapMode: ListView.SnapOneItem
orientation: Qt.Horizontal
model: 3
delegate: Item {
width: listView.width; height: listView.height
Text {
text: index
font.pixelSize: 0.9 * listView.height
anchors.centerIn: parent
}
}
onMovementEnded: listView.currentIndex = listView.indexAt(contentX, 0)
PageDots {
width: 0.25 * parent.width; height: 0.1 * parent.height
anchors.horizontalCenter: parent.horizontalCenter
anchors.bottom: parent.bottom
page: listView.currentIndex
pages: listView.count
}
}
Summary
You should now be familiar with how to create PageDots. Next time we'll cover Tabs. The source code can be downloaded here.